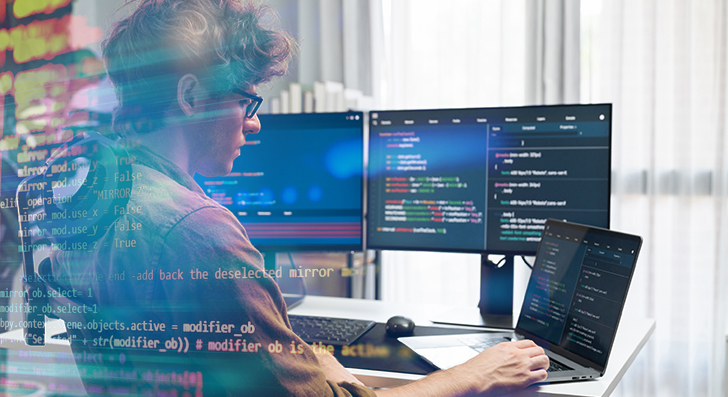
Scalability means your application can manage development—more people, a lot more information, and more targeted visitors—devoid of breaking. Like a developer, building with scalability in your mind saves time and worry afterwards. Listed here’s a clear and realistic guidebook that will help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really one thing you bolt on later—it should be part of the plan from the start. Many apps are unsuccessful whenever they expand fast due to the fact the first design and style can’t tackle the extra load. For a developer, you have to Consider early about how your procedure will behave under pressure.
Start off by designing your architecture for being adaptable. Avoid monolithic codebases the place all the things is tightly connected. Instead, use modular design and style or microservices. These designs crack your app into smaller sized, impartial pieces. Every module or provider can scale By itself without having impacting The complete system.
Also, take into consideration your database from working day just one. Will it need to handle one million buyers or simply a hundred? Choose the appropriate form—relational or NoSQL—dependant on how your data will develop. Program for sharding, indexing, and backups early, Even when you don’t need them yet.
Yet another crucial position is to stop hardcoding assumptions. Don’t produce code that only is effective less than present-day situations. Think of what would come about In the event your person foundation doubled tomorrow. Would your application crash? Would the databases slow down?
Use design and style designs that help scaling, like information queues or party-pushed devices. These support your app manage a lot more requests without having acquiring overloaded.
Whenever you Create with scalability in mind, you're not just preparing for fulfillment—you might be cutting down foreseeable future head aches. A nicely-planned method is easier to take care of, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the ideal Databases
Selecting the correct databases is usually a critical Section of developing scalable purposes. Not all databases are designed precisely the same, and using the Completely wrong one can slow you down or maybe bring about failures as your app grows.
Start by knowledge your info. Can it be hugely structured, like rows within a desk? If Indeed, a relational databases like PostgreSQL or MySQL is a superb in shape. They're potent with associations, transactions, and regularity. Additionally they support scaling tactics like study replicas, indexing, and partitioning to manage much more visitors and facts.
In case your facts is more versatile—like person action logs, products catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured info and might scale horizontally more simply.
Also, consider your go through and produce patterns. Have you been accomplishing a great deal of reads with much less writes? Use caching and read replicas. Do you think you're managing a heavy compose load? Check into databases which can deal with substantial produce throughput, and even celebration-centered information storage techniques like Apache Kafka (for momentary details streams).
It’s also smart to Believe forward. You may not need to have Highly developed scaling functions now, but picking a databases that supports them suggests you received’t have to have to modify afterwards.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your info according to your obtain styles. And normally observe databases general performance when you mature.
To put it briefly, the right databases relies on your application’s structure, velocity requires, And exactly how you hope it to mature. Acquire time to select correctly—it’ll preserve plenty of problems later.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, just about every smaller delay adds up. Improperly penned code or unoptimized queries can decelerate functionality and overload your program. That’s why it’s crucial that you Create effective logic from the start.
Get started by producing thoroughly clean, easy code. Avoid repeating logic and take away anything at all pointless. Don’t pick the most intricate Option if an easy one is effective. Maintain your functions small, targeted, and straightforward to test. Use profiling tools to uncover bottlenecks—areas where your code requires much too prolonged to run or works by using an excessive amount of memory.
Future, have a look at your databases queries. These typically slow factors down more than the code by itself. Be sure each question only asks for the info you really have to have. Stay away from Find *, which fetches every little thing, and in its place pick unique fields. Use indexes to speed up lookups. And keep away from carrying out a lot of joins, Particularly across significant tables.
In the event you detect the same info remaining requested over and over, use caching. Retail outlet the results briefly using resources like Redis or Memcached therefore you don’t really have to repeat costly operations.
Also, batch your databases functions after you can. Rather than updating a row one by one, update them in teams. This cuts down on overhead and would make your application more successful.
Make sure to exam with large datasets. Code and queries that perform wonderful with one hundred documents could possibly crash once they have to deal with 1 million.
In a nutshell, scalable apps are rapidly applications. Keep the code limited, your queries lean, and use caching when needed. These actions assist your application remain easy and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to deal with much more consumers plus much more targeted visitors. If all the things goes as a result of a person server, it will eventually quickly turn into a bottleneck. That’s wherever load balancing and caching are available. Both of these equipment aid keep your app fast, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of just one server undertaking every one of the perform, the load balancer routes customers to different servers dependant on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this very easy to put in place.
Caching is about storing information quickly so it could be reused rapidly. When buyers ask for the identical information again—like an item webpage or a profile—you don’t should fetch it from your databases whenever. You are able to provide it from your cache.
There's two widespread types of caching:
one. Server-side caching (like Redis or Memcached) merchants knowledge in memory for quick entry.
2. Customer-side caching (like browser caching or CDN caching) merchants static files near to the person.
Caching decreases databases load, improves pace, and makes your application more productive.
Use caching for things that don’t improve usually. And normally ensure your cache is current when information does adjust.
In short, load balancing and caching are straightforward but impressive resources. Jointly, they assist your app take care of extra customers, continue to be quick, and Get well from complications. If you plan to expand, you would like each.
Use Cloud and Container Equipment
To develop scalable purposes, you'll need equipment that permit your application grow effortlessly. That’s the place cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to buy hardware or guess long term capacity. When site visitors boosts, you could increase extra means with just some clicks or automatically using auto-scaling. When traffic drops, you can scale down to save money.
These platforms also give products and services like managed databases, storage, load balancing, and stability applications. You could center on making your application in place of taking care of infrastructure.
Containers are A different essential Device. A container packages your application and all the things it ought to run—code, libraries, settings—into one device. This causes it to be simple to maneuver your application among environments, from your notebook to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application makes use of numerous containers, applications like Kubernetes make it easier to control them. Kubernetes handles deployment, scaling, and recovery. If just one element of your application crashes, it restarts it instantly.
Containers also make it very easy to separate aspects of your app into providers. You can update or scale areas independently, that is perfect for efficiency and trustworthiness.
In a nutshell, using cloud and container equipment means you may scale quick, deploy quickly, and recover promptly when issues transpire. If you would like your application to grow without having limits, start out using these equipment early. They help you save time, decrease possibility, and help you remain centered on building, not repairing.
Watch Every thing
In case you don’t observe your application, you won’t know when factors go Completely wrong. Monitoring aids the thing is how your application is executing, place difficulties early, and make better choices as your app grows. It’s a essential Element of building scalable techniques.
Start off by monitoring essential metrics like CPU usage, memory, disk space, and response time. These let you know how your servers and companies are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this information.
Don’t just check your servers—observe your application much too. Keep an eye on how long it takes for users to load pages, how often errors occur, and exactly where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place inside your code.
Set up alerts for important problems. For example, if your reaction time goes higher than a Restrict or maybe a company goes down, you'll more info want to get notified straight away. This can help you deal with troubles quickly, usually prior to users even notice.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again before it results in true injury.
As your application grows, traffic and facts boost. With out checking, you’ll skip indications of difficulties till it’s much too late. But with the best resources set up, you remain on top of things.
In brief, checking aids you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about comprehension your system and making certain it works properly, even under pressure.
Ultimate Views
Scalability isn’t only for large corporations. Even little applications need a powerful Basis. By creating thoroughly, optimizing wisely, and using the ideal resources, you may Develop applications that mature easily devoid of breaking under pressure. Commence smaller, Believe massive, and Establish intelligent.